Fizz Buzz is a basic programming challenge that is commonly posed in software developer job interviews. Today in this tutorial, we will see how we can tackle this problem. We will first see the simple method to do it and later on, we will see some one-liner code to deal with it.
What is the Python Fizzbuzz?
Assume we have a number n. Then we have to display all numbers ranging from 1 to n in string representation, but there are few restrictions on how we can show those numbers
- If the number is divisible by three, substitute Fizz for the number.
- If the number is divisible by 5, substitute Buzz for the number.
- If the number is divisible by 3 and 5, substitute FizzBuzz for the integer.
Solution of python fizzbuzz
We will take the following measures to resolve this. − If a number is divisible by 3 and 5 both, print “FizzBuzz” otherwise, when the number is divisible by 3, print “Fizz” else, when the number is divisible by 5, print “Buzz” otherwise, write the number as a string.
Also read –> How to built hangman game with ASCII art in python
fizzbuzz python, fizzbuzz challenge python, shortest fizzbuzz python, fizzbuzz python hackerrank solution, fizzbuzz python solution
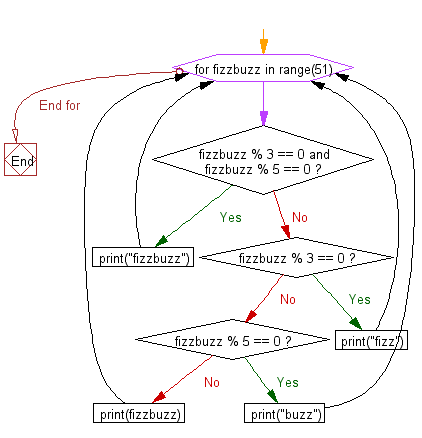
3 Ways to solve Python FizzBuzz in one line
So, we saw how we can solve fizzbuzz, but if you are challenged to solve it in one line then don’t worry we got you covered. I will show you three ways to solve it. The last two solutions are picked from Github.
Also read –> How to make your own audiobook from pdf using python
# fizzbuzz using list comprehension (easy) fizzbuzz = ['fizzbuzz' if v%3 == 0 and v%5 == 0 else 'fizz' if v % 3 == 0 else 'buzz' if v % 5 == 0 else v for v in range(1, 51)] print('First way\n',fizzbuzz) # fizzbuzz using list comprehension (intermediate) from github fizzbuzz = ["Fizz"*(i%3==0)+"Buzz"*(i%5==0) or str(i) for i in range(1, 51)] print('\n Second way\n',fizzbuzz) # fizzbuzz using lambda -(intermediate) from github fizzbuzz = list(map(lambda i: "Fizz"*(i%3==0)+"Buzz"*(i%5==0) or str(i), range(1,51))) print('\n Third way\n', fizzbuzz)
Try this on live code editor
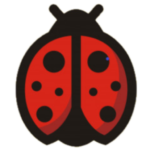
Data Scientist with 3+ years of experience in building data-intensive applications in diverse industries. Proficient in predictive modeling, computer vision, natural language processing, data visualization etc. Aside from being a data scientist, I am also a blogger and photographer.
- Aman Kumarhttps://buggyprogrammer.com/author/buggy5454/
- Aman Kumarhttps://buggyprogrammer.com/author/buggy5454/
- Aman Kumarhttps://buggyprogrammer.com/author/buggy5454/
- Aman Kumarhttps://buggyprogrammer.com/author/buggy5454/